As a data collection technician, navigating the intricate web of internet security is part of the daily grind. Cloudflare, a leading security provider, offers robust protection for websites, presenting challenges that must be overcome for successful data scraping. This guide will walk you through the process of using the Cloudflare API Gateway, focusing on how to bypass Cloudflare’s defenses, including the 5-second shield, WAF (Web Application Firewall) protection, and Turnstile CAPTCHA. By leveraging the Through Cloud API, you can seamlessly access your target websites without any barriers.
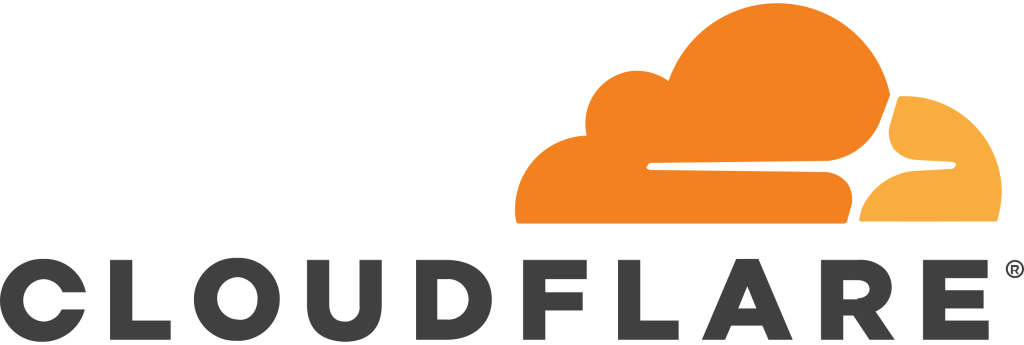
Understanding Cloudflare’s Security Measures
Cloudflare’s security suite is designed to protect websites from malicious activities. Here are the primary defenses you’ll encounter:
5-Second Shield: This JavaScript challenge adds a 5-second delay before granting access to ensure the request is legitimate.
WAF (Web Application Firewall): WAF filters and monitors HTTP requests to protect against attacks.
Turnstile CAPTCHA: A challenge-response test that differentiates human users from automated bots.
For a data collection technician, these measures can be significant hurdles. However, with the right tools and techniques, these defenses can be bypassed effectively.
Introducing Through Cloud API
The Through Cloud API is a comprehensive solution designed to help bypass Cloudflare’s security measures. It provides:
HTTP API: Facilitates direct interactions with websites.
Global Dynamic IP Proxy Service: Offers a vast pool of dynamic IP addresses, including residential and data center IPs from over 200 countries.
Customizable Request Parameters: Allows users to set Referer, User-Agent, and headless status, mimicking genuine browser behavior.
With the Through Cloud API, you can bypass Cloudflare’s defenses, ensuring seamless access to your target websites.
Step-by-Step Guide to Using Through Cloud API
Step 1: Register for Through Cloud API
Begin by registering for an account on the Through Cloud API platform. Visit the registration page, fill in the required details, and create your account. Upon registration, you will receive an API key, essential for accessing the API services.
Step 2: Setting Up Your Development Environment
Ensure your development environment is ready. This tutorial uses Python, a popular language for web scraping. Install the necessary libraries:
pip install requests
pip install beautifulsoup4
These libraries will help you make HTTP requests and parse HTML content.
Step 3: Bypassing the 5-Second Shield
The 5-second shield can be a major obstacle when accessing Cloudflare-protected websites. The Through Cloud API handles this challenge seamlessly. Here’s how to make a request using the Through Cloud API to bypass this shield:
import requests
api_key = ‘YOUR_API_KEY’
url = ‘https://example.com’
headers = {
‘Referer’: ‘https://example.com’,
‘User-Agent’: ‘Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36’,
}
params = {
‘api_key’: api_key,
‘url’: url,
}
response = requests.get(‘https://throughcloudapi.com/bypass’, headers=headers, params=params)
print(response.text)
In this example, a GET request is made to the Through Cloud API endpoint with the necessary headers and parameters. The API handles the 5-second shield, granting direct access to the website content.
Step 4: Navigating WAF Protection
WAF protection can block requests based on various criteria. Through Cloud API’s proxy service helps in rotating IPs and disguising requests to avoid detection. Here’s how to use it:
proxies = {
‘http’: ‘http://your-proxy-ip:port’,
‘https’: ‘https://your-proxy-ip:port’,
}
response = requests.get(‘https://example.com’, headers=headers, proxies=proxies)
print(response.text)
By using residential or data center proxies provided by Through Cloud, you can bypass WAF protection and ensure uninterrupted access to your target websites.
Step 5: Overcoming Turnstile CAPTCHA
Turnstile CAPTCHA is a significant barrier for automated systems. Through Cloud API provides a solution to bypass this challenge. Here’s an example of how to handle CAPTCHA challenges:
params = {
‘api_key’: api_key,
‘url’: ‘https://example.com/login’,
‘captcha’: ‘turnstile’,
}
response = requests.get(‘https://throughcloudapi.com/bypass’, headers=headers, params=params)
print(response.text)
This request instructs the API to handle the CAPTCHA challenge, allowing seamless access without manual intervention.
Step 6: Implementing Custom Request Parameters
To make your requests appear more human-like and avoid detection, customize various request parameters. Here’s how:
custom_headers = {
‘Referer’: ‘https://example.com’,
‘User-Agent’: ‘Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36’,
‘X-Requested-With’: ‘XMLHttpRequest’,
}
response = requests.get(‘https://example.com’, headers=custom_headers, proxies=proxies)
print(response.text)
By setting these headers, you mimic legitimate browser requests, reducing the likelihood of being flagged as a bot.
Step 7: Automating the Process
To automate data collection, you can write a script that periodically makes requests and processes the data. Here’s an example:
import time
from bs4 import BeautifulSoup
def fetch_data(url):
response = requests.get(url, headers=custom_headers, proxies=proxies)
if response.status_code == 200:
return response.text
return None
def parse_data(html):
soup = BeautifulSoup(html, ‘html.parser’)
# Extract data as needed
data = soup.find_all(‘div’, class_=’data-class’)
return data
def main():
url = ‘https://example.com’
while True:
html = fetch_data(url)
if html:
data = parse_data(html)
print(data)
time.sleep(60) # Wait for 60 seconds before the next request
if name == ‘main‘:
main()
This script fetches data from the target website every 60 seconds, parses it using BeautifulSoup, and prints the extracted data. Customize the parsing logic to suit your specific needs.
Step 8: Handling Errors and Exceptions
Web scraping is not always smooth sailing. Handle errors and exceptions gracefully to ensure your script runs reliably:
def fetch_data(url):
try:
response = requests.get(url, headers=custom_headers, proxies=proxies, timeout=10)
response.raise_for_status()
return response.text
except requests.RequestException as e:
print(f’Error fetching data: {e}’)
return None
By implementing error handling, you can manage network issues, request failures, and other potential problems effectively.
Step 9: Storing and Analyzing Data
Collected data is valuable only if it’s stored and analyzed properly. Use databases or file storage systems to save your data:
import csv
def save_data(data):
with open(‘data.csv’, ‘a’, newline=”) as file:
writer = csv.writer(file)
writer.writerow(data)
Example of saving parsed data
data = parse_data(html)
for item in data:
save_data([item.text])
In this example, we save the extracted data to a CSV file. You can use databases like SQLite, MongoDB, or any other storage system that fits your needs.
Real-Life Applications
E-commerce Price Monitoring
Imagine you’re tasked with monitoring prices on an e-commerce site. Using Through Cloud API, you can bypass Cloudflare’s defenses and collect price data at regular intervals:
url = ‘https://ecommerce-example.com/product-page’
html = fetch_data(url)
data = parse_data(html)
Extract and save price data
price = data.find(‘span’, class_=’price’).text
save_data([price])
By automating this process, you can maintain an up-to-date database of product prices.
Content Aggregation
If you’re building a news aggregator, Through Cloud API can help you gather content from multiple sources:
urls = [
‘https://news-site1.com’,
‘https://news-site2.com’,
‘https://news-site3.com’,
]
for url in urls:
html = fetch_data(url)
data = parse_data(html)
# Extract and save news headlines
headlines = [item.text for item in data.find_all(‘h1′, class_=’headline’)]
for headline in headlines:
save_data([headline])
This script collects headlines from multiple news websites, allowing you to create a comprehensive news feed.
Web Data Mining
For data scientists and researchers, accessing large datasets from various websites is crucial. Using Through Cloud API, you can automate the extraction of valuable data for analysis:
url = ‘https://data-source-example.com’
html = fetch_data(url)
data = parse_data(html)
Process and analyze the extracted data
processed_data = [process_item(item) for item in data]
analyze_data(processed_data)
This process enables efficient data mining and analysis, providing insights and trends that can drive decision-making.