In the ever-evolving realm of web scraping, Selenium stands out as a powerful tool for simulating human interactions with web pages. It allows developers to automate browsing, mimic human behavior, and collect data from websites that are otherwise difficult to scrape. However, as web security mechanisms, especially those employed by Cloudflare, become more advanced, scraping without detection has become increasingly challenging. This article delves into best practices and tips for preventing detection while using Selenium, focusing on how to bypass Cloudflare’s defenses effectively.
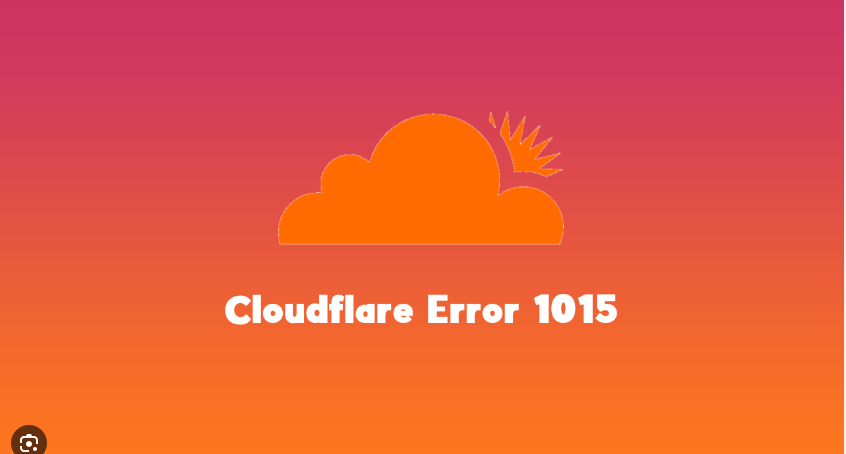
Understanding the Challenge: Cloudflare’s Defenses
Cloudflare is widely known for its robust security measures, which include a variety of anti-bot protections such as:
- Cloudflare WAF (Web Application Firewall): Filters and blocks suspicious activities.
- 5-Second Shield: Forces a delay, verifying the legitimacy of a request.
- Turnstile CAPTCHA: Presents a challenge that must be solved to continue.
These measures are designed to protect websites from malicious traffic but can also impede legitimate data collection efforts. As a data collection technician, overcoming these barriers without detection requires a combination of strategic practices and advanced tools, such as Through Cloud API.
Why Selenium?
Selenium is a popular choice for web scraping due to its ability to interact with web pages in a manner similar to human users. It can render JavaScript, handle dynamic content, and automate tasks in web browsers. Despite its advantages, Selenium alone may not be sufficient to bypass sophisticated defenses like those of Cloudflare. Enhancing Selenium’s capabilities with additional strategies and tools is essential for successful data collection.
Best Practices for Using Selenium
1. Mimic Human Behavior
One of the primary ways to prevent detection is to make your Selenium interactions indistinguishable from those of a human user. Here are some tips:
Randomize Actions
Avoid patterns that could be flagged as robotic behavior. This includes varying the timing of your clicks and scrolling, and introducing random delays between actions.
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
import random
driver = webdriver.Chrome()
driver.get('http://example.com')
# Random delay
time.sleep(random.uniform(1, 5))
search_box = driver.find_element_by_name('q')
search_box.send_keys('Selenium')
# Random delay
time.sleep(random.uniform(1, 5))
search_box.send_keys(Keys.RETURN)
Emulate Mouse Movements
Real users move their mouse in unpredictable ways. Using tools to simulate realistic mouse movements can help reduce detection.
from selenium.webdriver import ActionChains
action = ActionChains(driver)
element = driver.find_element_by_id('element-id')
# Simulate realistic mouse movement
action.move_to_element(element).perform()
2. Rotate User-Agents
User-Agent strings are sent with HTTP requests to identify the browser and device. Using a static User-Agent can make your bot easily detectable. Instead, rotate User-Agent strings to mimic different devices and browsers.
from selenium.webdriver.chrome.options import Options
options = Options()
user_agents = [
'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
]
options.add_argument(f"user-agent={random.choice(user_agents)}")
driver = webdriver.Chrome(options=options)
3. Use Proxies
To prevent IP-based blocking, use proxies to distribute your requests across multiple IP addresses. A reliable proxy service can help you avoid rate limiting and bans.
Through Cloud API provides a one-stop global dynamic data center/residential IP proxy service. It includes over 350 million dynamic IPs, which can rotate to prevent detection.
from selenium.webdriver.common.proxy import Proxy, ProxyType
proxy = Proxy()
proxy.proxy_type = ProxyType.MANUAL
proxy.http_proxy = "ip:port"
proxy.ssl_proxy = "ip:port"
capabilities = webdriver.DesiredCapabilities.CHROME
proxy.add_to_capabilities(capabilities)
driver = webdriver.Chrome(desired_capabilities=capabilities)
4. Handle Captchas
Cloudflare’s Turnstile CAPTCHA is a significant challenge for bots. While manual captcha solving might be necessary for some scrapers, automated solutions like Through Cloud API can bypass these captchas, ensuring uninterrupted scraping.
5. Maintain Session State
Keeping cookies and session data can help simulate a more realistic browsing experience and prevent frequent re-verification. Use Selenium to store and reuse session cookies.
# Save cookies
cookies = driver.get_cookies()
# Load cookies
for cookie in cookies:
driver.add_cookie(cookie)
6. Avoid Detection Mechanisms
Many websites employ techniques to detect Selenium-driven browsers. Use custom browser profiles and avoid detectable Selenium signatures.
Customize WebDriver Executable Path
Some anti-bot measures detect default Selenium signatures. Customizing the path to the WebDriver executable can sometimes help avoid detection.
python复制代码from selenium import webdriver
driver_path = "/path/to/custom/webdriver"
driver = webdriver.Chrome(executable_path=driver_path)
Hide WebDriver Property
You can use JavaScript to override properties that reveal the presence of WebDriver.
python复制代码driver.execute_script("Object.defineProperty(navigator, 'webdriver', {get: () => undefined})")
Integrating Through Cloud API
Through Cloud API complements Selenium by providing dynamic IP rotation and automated CAPTCHA bypass. It allows you to bypass Cloudflare’s 5-second shield, human verification, and WAF protection without being detected.
Setting Up Through Cloud API with Selenium
- Register and Obtain API Key: Sign up on the Through Cloud API platform to get your API key.
- Configure HTTP API: Integrate Through Cloud API with your Selenium script to handle Cloudflare’s security checks.
import requests
api_url = "https://api.throughcloud.com/bypass"
api_key = "your_api_key"
target_url = "http://targetwebsite.com"
headers = {
"Authorization": f"Bearer {api_key}",
"Referer": "http://targetwebsite.com",
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36"
}
response = requests.get(api_url, headers=headers, params={"url": target_url})
if response.status_code == 200:
data = response.json()
print("Data collected:", data)
else:
print("Failed to bypass Cloudflare.")
Use Dynamic Proxies
Through Cloud API’s dynamic proxies can be used with Selenium to further enhance your scraping efforts.
from selenium.webdriver.common.proxy import Proxy, ProxyType
proxy = Proxy()
proxy.proxy_type = ProxyType.MANUAL
proxy.http_proxy = "throughcloud_ip:port"
proxy.ssl_proxy = "throughcloud_ip:port"
capabilities = webdriver.DesiredCapabilities.CHROME
proxy.add_to_capabilities(capabilities)
driver = webdriver.Chrome(desired_capabilities=capabilities)
Real-World Scenarios
Scenario 1: Collecting Market Data
You are tasked with gathering competitive pricing data from various e-commerce websites. These sites use Cloudflare to protect their data. By integrating Through Cloud API with Selenium, you can bypass Cloudflare’s defenses and automate data collection, ensuring your company remains competitive.
Scenario 2: Monitoring Travel Deals
Your job is to track and analyze travel deals from multiple booking sites. These sites frequently update their security measures to block bots. Using Through Cloud API, you can bypass Cloudflare’s security checks, gather the latest deals, and provide valuable insights to your clients.
Ethical Considerations and Best Practices
While scraping, it’s essential to adhere to ethical guidelines and respect website terms of service. Overusing scraping techniques can lead to legal issues and damage the relationship between data collectors and website owners.
Respect Robots.txt
Always check a website’s robots.txt
file to understand its scraping policies and comply with them.
Rate Limiting
Implement rate limiting in your scraping scripts to avoid overwhelming the target servers and triggering security defenses.
import time
# Implement a delay between requests
time.sleep(2)
Conclusion
Preventing detection while using Selenium requires a combination of advanced techniques and strategic use of tools like Through Cloud API. By mimicking human behavior, rotating User-Agents, using proxies, and integrating Through Cloud API’s advanced features, you can effectively bypass Cloudflare’s defenses, including the 5-second shield, WAF protection, and Turnstile CAPTCHA. This integration not only enhances your scraping capabilities but also ensures a smoother, more efficient data collection process.
As web security continues to evolve, staying ahead of detection mechanisms is crucial. Through Cloud API, combined with best practices for Selenium, provides a robust solution for data collection technicians seeking to navigate and overcome Cloudflare’s formidable barriers. This approach empowers you to collect valuable data while maintaining the integrity and effectiveness of your web scraping efforts.
4o