When working as a data collection technician, one of the crucial aspects of web scraping with Selenium is ensuring that the page has fully loaded before proceeding with any actions. Premature actions can lead to incomplete data, broken scripts, or, worse, detection by advanced security measures such as those employed by Cloudflare. In this comprehensive guide, we’ll explore various strategies for waiting for page load completion in Selenium, and integrate these with techniques to bypass Cloudflare’s security mechanisms using Through Cloud API.
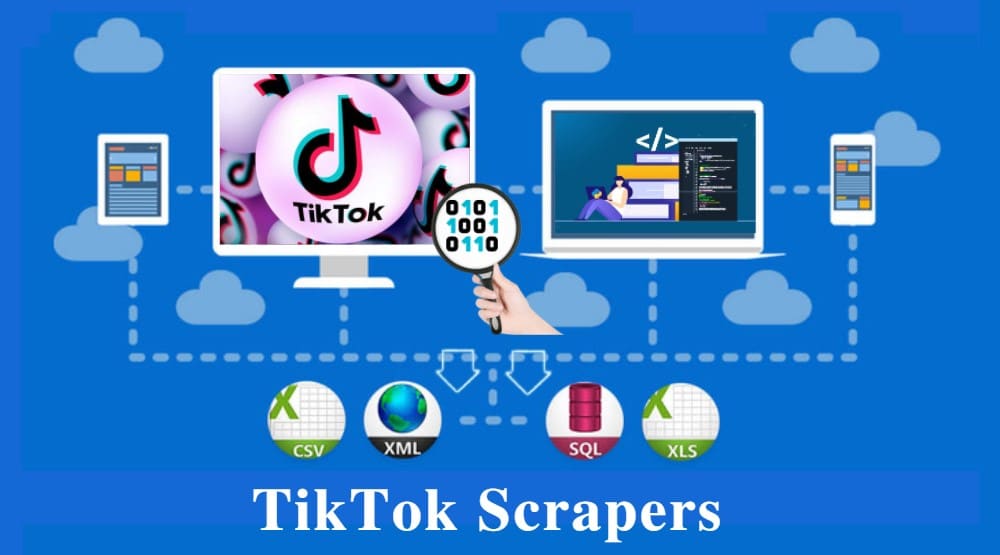
The Challenge of Page Load Completion
Web pages today are dynamic and often load content asynchronously, which can pose challenges for web scraping. Traditional waiting methods might not always work, leading to errors or incomplete data collection. Advanced security systems like Cloudflare further complicate matters by adding extra layers of verification, such as the 5-second shield and Turnstile CAPTCHA.
Basic Waiting Techniques in Selenium
1. Implicit Waits
Implicit waits set a global wait time for the entire WebDriver session. If the element is not found within the specified time, an exception is thrown. This is useful for dealing with moderately slow loading times but can be inefficient for dynamically loaded content.
from selenium import webdriver
driver = webdriver.Chrome()
driver.implicitly_wait(10) # Wait up to 10 seconds for elements to appear
driver.get('http://example.com')
2. Explicit Waits
Explicit waits allow you to wait for a specific condition to occur before proceeding. This can be more efficient than implicit waits, especially for handling asynchronous content.
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver = webdriver.Chrome()
driver.get('http://example.com')
# Wait for a specific element to be visible
element = WebDriverWait(driver, 10).until(
EC.visibility_of_element_located((By.ID, 'element-id'))
)
3. Fluent Waits
Fluent waits are similar to explicit waits but allow for more customization, including polling intervals and the ability to ignore specific exceptions.
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.common.exceptions import NoSuchElementException
wait = WebDriverWait(driver, 10, poll_frequency=1, ignored_exceptions=[NoSuchElementException])
element = wait.until(
EC.visibility_of_element_located((By.ID, 'element-id'))
)
Advanced Waiting Strategies
4. Waiting for JavaScript Execution
Web pages often use JavaScript to load additional content. Waiting for the document’s ready state can ensure that the initial page load is complete.
python复制代码# Wait for the document to be fully loaded
driver.execute_script("return document.readyState") == "complete"
5. Waiting for AJAX Calls to Complete
Many modern web applications use AJAX to load data asynchronously. Waiting for these calls to complete can be tricky but is essential for accurate data collection.
# Wait for all AJAX requests to complete
def wait_for_ajax(driver):
wait = WebDriverWait(driver, 10)
wait.until(lambda driver: driver.execute_script('return jQuery.active') == 0)
wait_for_ajax(driver)
6. Monitoring Network Requests
Using browser tools to monitor network requests can help you wait for specific resources to load, ensuring that all necessary data has been retrieved.
# Example using Chrome DevTools Protocol to monitor network requests (pseudo-code)
# Note: Requires additional setup and libraries
Integrating Through Cloud API for Bypassing Cloudflare
Cloudflare’s defenses, such as the 5-second shield and WAF protection, can significantly hinder scraping efforts. Through Cloud API provides a robust solution for bypassing these barriers, ensuring uninterrupted access to web content.
Using Through Cloud API with Selenium
Through Cloud API offers an HTTP API and dynamic IP proxy service that can bypass Cloudflare’s security measures, including Turnstile CAPTCHA. Here’s how you can integrate Through Cloud API with Selenium for a seamless scraping experience.
Setting Up Through Cloud API
- Register and Obtain API Key: Sign up on Through Cloud API’s platform to get your API key.
- Configure HTTP API: Use the API to bypass Cloudflare’s verification processes.
import requests
api_url = "https://api.throughcloud.com/bypass"
api_key = "your_api_key"
target_url = "http://targetwebsite.com"
headers = {
"Authorization": f"Bearer {api_key}",
"Referer": target_url,
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36"
}
response = requests.get(api_url, headers=headers, params={"url": target_url})
if response.status_code == 200:
print("Successfully bypassed Cloudflare")
else:
print("Failed to bypass Cloudflare")
- Use Dynamic Proxies: Integrate the dynamic proxy service to rotate IPs and avoid detection.
from selenium.webdriver.common.proxy import Proxy, ProxyType
proxy = Proxy()
proxy.proxy_type = ProxyType.MANUAL
proxy.http_proxy = "throughcloud_ip:port"
proxy.ssl_proxy = "throughcloud_ip:port"
capabilities = webdriver.DesiredCapabilities.CHROME
proxy.add_to_capabilities(capabilities)
driver = webdriver.Chrome(desired_capabilities=capabilities)
Setting Browser Fingerprints
To further evade detection, Through Cloud API allows you to set custom browser fingerprints, including Referer, User-Agent, and headless states.
from selenium.webdriver.chrome.options import Options
options = Options()
options.add_argument("user-agent=your_custom_user_agent")
options.add_argument("referer=http://targetwebsite.com")
options.headless = True # Run in headless mode for efficiency
driver = webdriver.Chrome(options=options)
Real-World Applications and Strategies
Scenario 1: Scraping Dynamic Content
When scraping a website that loads content dynamically through AJAX, combining Selenium’s AJAX waiting strategies with Through Cloud API’s CAPTCHA bypass can streamline the data collection process.
- Wait for AJAX Calls: Use custom waiting functions to ensure all data is loaded.
- Bypass Cloudflare: Utilize Through Cloud API to handle any Cloudflare verification challenges.
Scenario 2: Automated Data Collection for Competitive Analysis
In competitive markets, staying ahead requires real-time data. Using Selenium with Through Cloud API allows for:
- Automated Scraping: Set up scripts to collect data at regular intervals without manual intervention.
- Bypassing Defenses: Ensure your bots aren’t blocked by Cloudflare’s defenses, maintaining a steady flow of data.
Scenario 3: Large-Scale Web Data Mining
For projects requiring large volumes of data, efficiency and reliability are paramount.
- Dynamic IPs: Through Cloud API’s dynamic proxies help distribute requests and avoid rate limiting.
- Efficient Waiting: Implementing advanced waiting strategies ensures data integrity and completeness.
Personal Insights and Recommendations
Balance Between Automation and Manual Monitoring
While automation is the goal, manual monitoring of your scraping scripts can provide insights into new challenges and evolving defenses. Regularly reviewing logs and outputs helps in fine-tuning strategies.
Ethical Considerations
Scraping should always respect the website’s terms of service and ethical guidelines. Use techniques responsibly, and avoid actions that could disrupt the website’s normal operations.
Staying Updated
The field of web scraping and bypassing security measures is dynamic. Keeping up with the latest developments, tools, and best practices is crucial. Engage with communities and forums to stay informed.
Conclusion
Waiting for page load completion in Selenium is crucial for effective and accurate data scraping. By employing a combination of basic and advanced waiting strategies, you can ensure that your scripts interact with fully loaded pages, minimizing errors and maximizing data integrity. Integrating Through Cloud API further enhances your capabilities by bypassing Cloudflare’s sophisticated defenses, allowing seamless access to protected content.
As a data collection technician, mastering these techniques not only improves your scraping efficiency but also equips you with the tools to handle increasingly complex web environments. By balancing automation with ethical considerations and continuous learning, you can navigate the challenges of modern web scraping with confidence and precision.