As a data collection technician, navigating the digital landscape often feels like a constant battle against web defenses designed to block our every move. Among these defenses, Cloudflare’s Bot Challenge, including its notorious 5-second shield and CAPTCHA, stands as one of the most formidable barriers. When using Selenium for web scraping, encountering Cloudflare’s protections can be a source of significant frustration and delay. However, with the right strategies and tools, such as Through Cloud API, it is possible to bypass Cloudflare’s defenses and achieve smooth access to your target websites.
In this comprehensive tutorial, we’ll explore how to effectively use Selenium and Through Cloud API to overcome Cloudflare’s obstacles, ensuring seamless data collection. We’ll delve into practical solutions, code snippets, and advanced configurations to bypass Cloudflare’s anti-bot mechanisms, including its Web Application Firewall (WAF) protections.
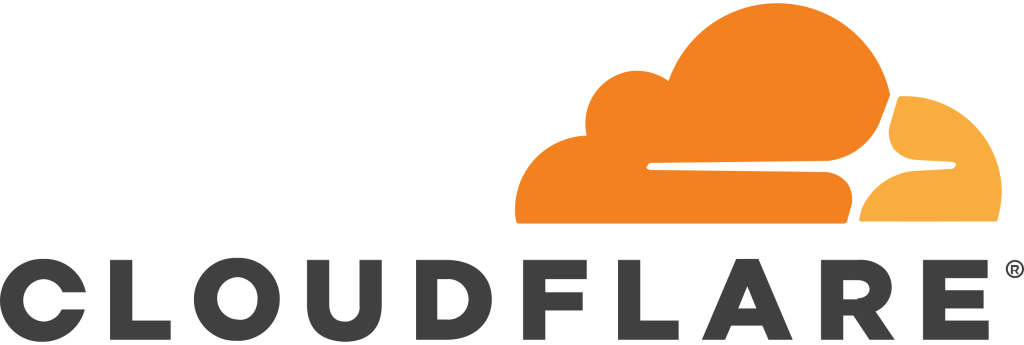
Understanding Cloudflare’s Challenges
The 5-Second Shield
Cloudflare’s 5-second shield is a waiting period that requires browsers to process JavaScript challenges before granting access. This shield is designed to detect automated bots by delaying the response time and analyzing browser behavior.
Turnstile CAPTCHA
Turnstile CAPTCHA is another layer of defense, presenting users with a challenge that requires human interaction. This often involves solving puzzles, selecting images, or performing actions that automated bots struggle with.
Web Application Firewall (WAF) Protections
Cloudflare’s WAF is a robust security feature that filters and monitors HTTP requests to block potentially harmful traffic. It inspects request headers, payloads, and behavior to identify and mitigate threats, often flagging automated scraping activities as suspicious.
Why Selenium Struggles with Cloudflare
Selenium, a popular tool for browser automation, mimics human interactions with web pages. However, it often gets flagged by Cloudflare’s defenses due to its predictable patterns and lack of human-like browsing behavior. The challenges include:
- JavaScript Execution Issues: Selenium might struggle with Cloudflare’s JavaScript challenges, leading to incomplete or incorrect page loads.
- Detection of Automation Patterns: Cloudflare’s algorithms can identify and block patterns typical of automated tools, including Selenium.
- Inability to Solve CAPTCHAs: Selenium cannot handle interactive CAPTCHAs that require user inputs, stalling the scraping process.
Overcoming Cloudflare with Through Cloud API
Through Cloud API is a versatile tool that offers solutions for bypassing Cloudflare’s defenses, including the 5-second shield, Turnstile CAPTCHA, and WAF protections. It provides an HTTP API and a global dynamic IP proxy service, enabling smooth access to target websites without the common hurdles faced by Selenium alone.
Key Features of Through Cloud API:
- Bypass Cloudflare’s 5-Second Shield: Skips the delay by handling JavaScript challenges externally.
- Circumvent Turnstile CAPTCHA: Solves or bypasses CAPTCHAs, allowing automated tools to proceed without interruption.
- Cloudflare WAF Bypass: Evades WAF protections to ensure requests are not flagged or blocked.
- HTTP API and Proxy Services: Offers both direct API access and dynamic IP proxy services for flexible integration.
- Customizable Browser Fingerprint: Allows setting of Referer, User-Agent, and headless status, mimicking real browser behavior to avoid detection.
Practical Steps to Bypass Cloudflare with Selenium and Through Cloud API
Let’s dive into a step-by-step approach to integrating Selenium with Through Cloud API for effective Cloudflare bypass.
Step 1: Set Up Through Cloud API
- Register for Through Cloud API: Visit the Through Cloud API website and sign up for an account. This will provide you with access to the API and proxy services.
- Generate API Keys: Once registered, generate your API keys from the Through Cloud dashboard. These keys are essential for authenticating your requests.
- Configure API Access: Set up the API endpoints, request parameters, and response handling as per the Through Cloud documentation. This setup will be used to integrate with your Selenium scripts.
Step 2: Install Selenium and Required Libraries
Ensure you have Selenium and other necessary Python libraries installed:
bash复制代码pip install selenium requests
Step 3: Integrate Through Cloud API with Selenium
Here’s how to integrate Through Cloud API into your Selenium workflow:
- Initialize Through Cloud API: Use the HTTP API to handle initial Cloudflare challenges before passing control to Selenium.
- Proxy Configuration: Configure Selenium to use Through Cloud’s dynamic IP proxy to rotate IP addresses, reducing the risk of being flagged by Cloudflare.
- Custom Headers and User-Agent: Customize request headers and User-Agent strings to emulate real browser behavior and avoid detection.
from selenium import webdriver
from selenium.webdriver.common.proxy import Proxy, ProxyType
# Configure Through Cloud API Proxy
proxy = Proxy()
proxy.proxy_type = ProxyType.MANUAL
proxy.http_proxy = "http://your-proxy-address:port"
proxy.ssl_proxy = "http://your-proxy-address:port"
# Set up Selenium with Proxy
capabilities = webdriver.DesiredCapabilities.CHROME
proxy.add_to_capabilities(capabilities)
options = webdriver.ChromeOptions()
options.add_argument("user-agent=YourCustomUserAgentString")
options.add_argument("--headless")
driver = webdriver.Chrome(desired_capabilities=capabilities, options=options)
# Access the target website
driver.get("https://target-website.com")
Step 4: Handle JavaScript Challenges
Use Through Cloud API to process JavaScript challenges externally:
- Send Request via Through Cloud API: Use
requests
library to send an initial request to the target website through Through Cloud API.
python复制代码import requests
api_url = "https://throughcloud-api.com/bypass"
payload = {
"url": "https://target-website.com",
"headers": {"User-Agent": "YourCustomUserAgentString"}
}
response = requests.get(api_url, params=payload)
cookies = response.cookies
- Transfer Cookies to Selenium: Transfer the session cookies obtained from Through Cloud API to Selenium to maintain the session.
# Set Cookies in Selenium
for cookie in cookies:
driver.add_cookie({"name": cookie.name, "value": cookie.value})
# Refresh Selenium to continue with authenticated session
driver.refresh()
Step 5: Solving CAPTCHAs
When encountering CAPTCHAs, Through Cloud API can handle them or provide a method to bypass:
- CAPTCHA Handling via Through Cloud API: Automatically solve or bypass CAPTCHAs using Through Cloud’s capabilities.
# Example API call to bypass CAPTCHA
captcha_url = "https://throughcloud-api.com/captcha-bypass"
captcha_payload = {
"url": "https://target-website.com/captcha",
"headers": {"User-Agent": "YourCustomUserAgentString"}
}
captcha_response = requests.get(captcha_url, params=captcha_payload)
- Use CAPTCHA Tokens: Inject the CAPTCHA solution or bypass token into the Selenium session.
# Inject CAPTCHA solution in Selenium
driver.execute_script("document.querySelector('input[name=captcha-token]').value = 'bypass-token';")
Step 6: Navigating Cloudflare’s WAF
To bypass Cloudflare’s WAF protections, use Through Cloud API’s WAF bypass capabilities:
- Send Requests via Through Cloud API: Funnel your HTTP requests through Through Cloud API to evade WAF detection.
waf_url = "https://throughcloud-api.com/waf-bypass"
waf_payload = {
"url": "https://target-website.com",
"headers": {"User-Agent": "YourCustomUserAgentString"}
}
waf_response = requests.get(waf_url, params=waf_payload)
- Handle Responses: Process the response data and cookies, ensuring that your Selenium session remains consistent with the API session.
python复制代码# Set WAF-bypassed cookies in Selenium
for cookie in waf_response.cookies:
driver.add_cookie({"name": cookie.name, "value": cookie.value})
# Continue with Selenium operations
driver.refresh()
Insights and Perspectives
Embracing Flexibility and Adaptation
One of the key lessons in bypassing Cloudflare’s defenses is the need for flexibility and adaptation. Static approaches often fail in the dynamic landscape of web security. By leveraging Through Cloud API, you gain the flexibility to handle evolving security measures, from JavaScript challenges to CAPTCHA and WAF protections.
Balancing Automation with Ethics
While it is technically feasible to bypass security measures, it’s crucial to approach web scraping with ethical considerations. Respect the terms of service of the websites you interact with, and ensure your activities are compliant with legal standards. Automation should be used responsibly to avoid misuse and ensure the integrity of the digital ecosystem.
Continuous Learning and Optimization
Web scraping and data collection are fields that require continuous learning and optimization. As security measures evolve, so too must your techniques and tools. Staying informed about the latest developments in web security and scraping technologies is essential for maintaining effective and compliant practices.