In the realm of web scraping and data collection, Cloudflare stands as a formidable gatekeeper, implementing a suite of anti-crawling defenses designed to protect websites from automated access. For data collection technicians, this presents significant challenges: the 5-second shield, Turnstile CAPTCHA, and Web Application Firewall (WAF) protections that thwart attempts to gather data seamlessly. Navigating these barriers requires sophisticated tools and strategies. This tutorial explores the best tools and methods for bypassing Cloudflare, offering insights and practical advice for integrating these techniques into your data collection workflows.
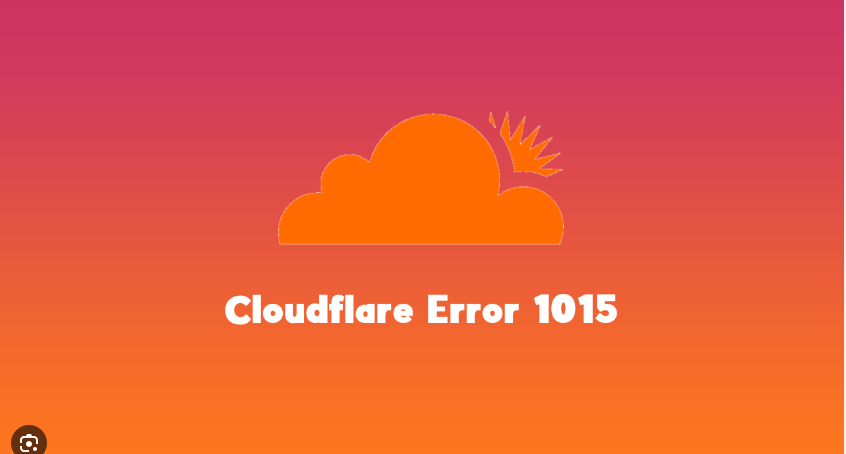
Understanding Cloudflare’s Defenses
Before diving into the tools, it’s crucial to understand the mechanisms Cloudflare employs:
- 5-Second Shield: A challenge page that delays access, giving the impression of a loading period to verify human interaction.
- Turnstile CAPTCHA: A CAPTCHA system designed to differentiate between human users and bots.
- WAF Protection: Web Application Firewall that filters and blocks malicious traffic, preventing many automated scripts from accessing content.
These layers create a multi-faceted defense that can be difficult to bypass without the right tools.
The Through Cloud API: A Comprehensive Solution
Among the various tools available, the Through Cloud API stands out for its robust capabilities. Designed to bypass Cloudflare’s defenses, this tool integrates seamlessly into data collection processes by offering an HTTP API and a built-in one-stop global dynamic IP proxy service. Here’s a breakdown of its features and how to use them effectively.
Key Features of Through Cloud API
- Bypass 5-Second Shield: Avoid the delay and access content without waiting.
- Cloudflare WAF Bypass: Navigate through Cloudflare’s firewall protections.
- Circumvent Turnstile CAPTCHA: Automatically bypass CAPTCHA verifications.
- Global Dynamic IP Proxy: Access over 350 million city-level dynamic IPs across more than 200 countries.
- Customization: Set Referer, browser User-Agent, and headless status, and configure browser fingerprint device features.
Setting Up Through Cloud API
- Register for an Account: Begin by creating an account on the Through Cloud API website.
- Use the Code Generator: Test the API’s ability to bypass Cloudflare defenses using the code generator.
- Integrate with Your Code: Incorporate the API into your Python scripts for automated data collection.
- Debug and Optimize: Adjust your scripts as needed to handle various scenarios and ensure seamless operation.
- Select a Plan: Choose a plan that meets your data collection requirements and budget.
Practical Implementation
Here’s how you can implement the Through Cloud API in a typical data collection scenario:
import requests
# Example function to bypass Cloudflare using Through Cloud API
def bypass_cloudflare(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://example.com'
}
response = requests.get(
'https://api.throughcloud.com/bypass',
headers=headers,
params={'url': url}
)
return response.json()
# Example usage
data = bypass_cloudflare('https://targetwebsite.com')
print(data)
This script demonstrates a simple method for making requests through the Through Cloud API, allowing you to bypass Cloudflare protections seamlessly.
Exploring Other Tools and Techniques
While Through Cloud API provides a powerful solution, other tools and techniques can complement your data collection strategy. Each has its strengths and is suited to different scenarios.
Residential Proxies
Residential proxies route your requests through IPs assigned to real residential addresses, reducing the likelihood of being blocked. Services like Luminati and Smartproxy offer extensive proxy networks that can be useful in avoiding detection.
Advantages:
- Mimics real user behavior.
- Reduces risk of IP bans.
Example:
from requests import Session
# Using Smartproxy for residential IPs
session = Session()
proxies = {
'http': 'http://user:[email protected]:port',
'https': 'http://user:[email protected]:port'
}
session.proxies.update(proxies)
response = session.get('https://targetwebsite.com')
print(response.text)
Headless Browsers
Headless browsers, like Puppeteer or Selenium, allow you to control a web browser via code without displaying a user interface. They can interact with JavaScript-heavy websites and handle CAPTCHA challenges by simulating user actions.
Advantages:
- Capable of handling dynamic content.
- Can execute JavaScript.
Example:
from selenium import webdriver
# Using Selenium for headless browsing
options = webdriver.ChromeOptions()
options.add_argument('headless')
driver = webdriver.Chrome(options=options)
driver.get('https://targetwebsite.com')
content = driver.page_source
print(content)
driver.quit()
Anti-CAPTCHA Services
Anti-CAPTCHA services like 2Captcha and AntiCaptcha provide APIs that can solve CAPTCHAs for you, integrating with headless browsers or other tools to streamline the data collection process.
Advantages:
- Automates CAPTCHA solving.
- Integrates with various scraping tools.
Example:
import requests
# Using 2Captcha to solve CAPTCHA
api_key = 'YOUR_API_KEY'
captcha_id = requests.post('http://2captcha.com/in.php', data={
'method': 'userrecaptcha',
'googlekey': 'SITE_KEY',
'pageurl': 'https://targetwebsite.com',
'key': api_key
}).text.split('|')[1]
# Check the CAPTCHA solving status
result = requests.get(f'http://2captcha.com/res.php?key={api_key}&action=get&id={captcha_id}').text
while 'CAPCHA_NOT_READY' in result:
result = requests.get(f'http://2captcha.com/res.php?key={api_key}&action=get&id={captcha_id}').text
solution = result.split('|')[1]
print(f'CAPTCHA Solution: {solution}')
Combining Tools for Optimal Results
To effectively bypass Cloudflare, a multi-faceted approach often yields the best results. Here’s how you can combine different tools and techniques:
- Initial Request via Proxies: Start with residential proxies to minimize detection.
- Handle Dynamic Content: Use headless browsers to interact with JavaScript-heavy pages.
- Automate CAPTCHA Solving: Integrate anti-CAPTCHA services to handle Turnstile CAPTCHA.
- Refine with Through Cloud API: Use the Through Cloud API for bypassing Cloudflare’s specific defenses and accessing content without delays.
Example Workflow
Let’s put together a workflow that incorporates these elements:
from selenium import webdriver
import requests
def get_page_content(url, captcha_solution=None):
# Set up proxies and headers
proxies = {
'http': 'http://user:[email protected]:port',
'https': 'http://user:[email protected]:port'
}
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
# Initial request through proxy
response = requests.get(url, proxies=proxies, headers=headers)
# Handle CAPTCHA if present
if 'CAPTCHA' in response.text and captcha_solution:
response = requests.post(
url,
data={'g-recaptcha-response': captcha_solution},
proxies=proxies,
headers=headers
)
return response.text
def bypass_cloudflare_and_collect(url):
# Use headless browser for dynamic content
options = webdriver.ChromeOptions()
options.add_argument('headless')
driver = webdriver.Chrome(options=options)
driver.get(url)
page_content = driver.page_source
driver.quit()
# Combine with Through Cloud API
cloud_api_response = requests.get(
'https://api.throughcloud.com/bypass',
params={'url': url},
headers={'User-Agent': 'Your-User-Agent'}
).json()
return cloud_api_response, page_content
# Usage
url = 'https://targetwebsite.com'
captcha_solution = 'solved_captcha_response'
page_content = get_page_content(url, captcha_solution)
cloudflare_data, dynamic_content = bypass_cloudflare_and_collect(url)
print('Static Page Content:', page_content)
print('Dynamic Content:', dynamic_content)
print('Through Cloud API Data:', cloudflare_data)
This example demonstrates a combined approach: using proxies for initial requests, handling dynamic content with a headless browser, solving CAPTCHA challenges, and leveraging Through Cloud API for effective Cloudflare bypassing.
Best Practices and Considerations
Respect Target Sites
While bypassing defenses is technically feasible, it’s essential to respect the terms of service and legal boundaries of target sites. Ethical data collection practices should always be a priority.
Optimize Request Rates
High request rates can raise red flags and lead to IP bans. Implement rate limiting and random delays to mimic human browsing patterns and avoid detection.