As a data collection technician, navigating the web’s protective barriers is a crucial skill. Cloudflare, with its Web Application Firewall (WAF), 5-second shield, and CAPTCHA mechanisms, stands as one of the most formidable gatekeepers. However, understanding and utilizing strategies to bypass these security features can unlock a wealth of data. This guide will delve into these strategies, with a focus on practical methods and tools such as Through Cloud API.
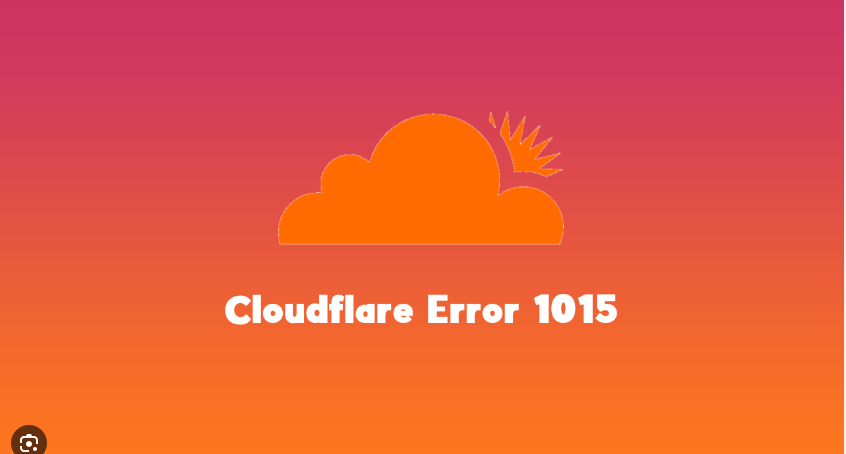
Understanding Cloudflare’s Security Features
Before diving into the bypass strategies, it’s essential to understand what you’re up against:
1. Cloudflare’s 5-Second Shield
This mechanism presents a brief delay page to all visitors, verifying if they are human by running JavaScript challenges. It aims to prevent bots from accessing the site while allowing human visitors through after a short wait.
2. Web Application Firewall (WAF)
Cloudflare’s WAF protects against malicious attacks by filtering and monitoring HTTP traffic between a web application and the Internet. It identifies and blocks common attack vectors such as SQL injection, XSS, and malicious bots.
3. Turnstile CAPTCHA
Cloudflare’s CAPTCHA requires users to prove they are human, typically by solving visual challenges. It’s designed to stop automated access and ensure that only legitimate users can proceed.
Strategies for Bypassing Cloudflare
1. Leveraging Through Cloud API
Through Cloud API offers a comprehensive solution for bypassing Cloudflare’s WAF, 5-second shield, and CAPTCHA. It provides an HTTP API and a global high-speed S5 dynamic IP proxy service, which are crucial in mimicking human-like behavior and accessing protected content.
Setting Up Through Cloud API
To get started with Through Cloud API:
- Register for an Account: Sign up on their website.
- Configure Your Requests: Use the code generator to test and configure your target URLs.
- Integrate API: Incorporate Through Cloud API into your data collection scripts.
Example Configuration:
curl -X POST https://api.throughcloud.com/v1/bypass \
-H "Content-Type: application/json" \
-H "User-Agent: your-user-agent" \
-d '{
"url": "https://target-website.com",
"method": "GET",
"headers": {
"Referer": "https://example.com"
},
"body": "{}"
}'
Using Dynamic Proxies
Through Cloud API provides access to over 350 million dynamic IPs globally. This diversity helps in rotating IPs, making it difficult for Cloudflare to detect and block the requests.
Example of Dynamic Proxy Usage:
const browser = await puppeteer.launch({
headless: true,
args: ['--proxy-server=https://proxy.throughcloud.com:8080']
});
2. Emulating Human Behavior
To bypass Cloudflare’s security features, emulating human-like behavior in your requests is crucial. This includes:
1. Mimicking User Actions
Automate actions such as mouse movements, scrolling, and clicks to simulate a human user.
Example with Puppeteer:
await page.goto('https://target-website.com');
await page.mouse.move(100, 200);
await page.mouse.click(100, 200);
2. Randomizing Delays
Introduce random delays between actions to mimic natural browsing behavior.
Example:
await page.waitForTimeout(Math.floor(Math.random() * 1000) + 500);
3. Managing Browser Fingerprints
Cloudflare tracks various aspects of your browser’s fingerprint, such as User-Agent, Referer, and headless status. By managing these fingerprints, you can make your automated requests appear more legitimate.
Setting User-Agent and Referer
await page.setExtraHTTPHeaders({
'User-Agent': 'your-user-agent',
'Referer': 'https://example.com'
});
Bypassing Headless Detection
Some websites can detect headless browsers. Tools like Puppeteer Stealth can help mask headless status.
Example with Puppeteer Stealth:
const puppeteer = require('puppeteer-extra');
const StealthPlugin = require('puppeteer-extra-plugin-stealth');
puppeteer.use(StealthPlugin());
const browser = await puppeteer.launch({
headless: false
});
4. Handling CAPTCHAs
While Through Cloud API can bypass many CAPTCHA challenges, there may be scenarios where you need additional strategies.
Using CAPTCHA Solving Services
Integrate CAPTCHA solving services that provide human or automated solving capabilities.
Example with 2Captcha:
const axios = require('axios');
async function solveCaptcha(sitekey, pageUrl) {
const response = await axios.post('http://2captcha.com/in.php', {
key: 'your-2captcha-api-key',
method: 'userrecaptcha',
googlekey: sitekey,
pageurl: pageUrl
});
return response.data;
}
5. Rotating IPs and Avoiding Detection
Constantly rotating IP addresses can help avoid detection and blocking. Through Cloud API’s dynamic IP service is particularly useful here, but you can also implement your own rotation logic.
Example of IP Rotation:
const proxyList = ['http://proxy1', 'http://proxy2', 'http://proxy3'];
let currentProxy = 0;
async function getNextProxy() {
currentProxy = (currentProxy + 1) % proxyList.length;
return proxyList[currentProxy];
}
6. Adapting to Rate Limiting
Cloudflare employs rate limiting to restrict the number of requests from a single IP. Managing your request rate is essential to avoid triggering these limits.
Implementing Rate Limiting:
javascript复制代码const rateLimit = 1000; // 1 request per second
async function makeRequest(url) {
// Implement your request logic here
await new Promise(resolve => setTimeout(resolve, rateLimit));
}
7. Using Browser Automation Tools
Browser automation tools like Puppeteer, Selenium, or Playwright can help bypass Cloudflare’s challenges by automating interactions within a real browser environment.
Example with Puppeteer:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
await page.goto('https://target-website.com');
// Perform actions here
await browser.close();
})();
8. Employing Machine Learning for Behavior Analysis
Machine learning can be used to analyze and mimic user behavior patterns more accurately, making it harder for Cloudflare to distinguish between bots and humans.
Example Approach:
- Collect User Interaction Data: Track real user interactions to gather data on mouse movements, typing patterns, etc.
- Train a Model: Use this data to train a model that predicts human-like behavior.
- Integrate the Model: Apply this model in your automation scripts to simulate realistic interactions.
Practical Application: Bypassing Cloudflare with Through Cloud API
Let’s apply these strategies to a real-world scenario. Suppose you’re tasked with collecting data from an e-commerce site protected by Cloudflare. Here’s a step-by-step approach using Through Cloud API:
Step 1: Setting Up
Register for a Through Cloud API account and configure your settings. This setup provides access to dynamic IPs and bypass mechanisms.
Step 2: Implementing Data Collection
Use Puppeteer along with Through Cloud API to automate the data collection process.
const puppeteer = require('puppeteer-extra');
const StealthPlugin = require('puppeteer-extra-plugin-stealth');
puppeteer.use(StealthPlugin());
(async () => {
const browser = await puppeteer.launch({
headless: true,
args: ['--proxy-server=https://proxy.throughcloud.com:8080']
});
const page = await browser.newPage();
await page.goto('https://target-ecommerce-site.com');
// Interact with the page, collect data
await browser.close();
})();
Step 3: Managing Requests and Avoiding Detection
Integrate IP rotation and rate limiting in your script to avoid being flagged by Cloudflare.
const proxyList = ['http://proxy1', 'http://proxy2', 'http://proxy3'];
let currentProxy = 0;
async function makeRequestWithRotation(url) {
const proxy = proxyList[currentProxy];
currentProxy = (currentProxy + 1) % proxyList.length;
// Configure Puppeteer with the new proxy
const browser = await puppeteer.launch({
headless: true,
args: [`--proxy-server=${proxy}`]
});
const page = await browser.newPage();
await page.goto(url);
// Perform actions here
await browser.close();
}
setInterval(() => {
makeRequestWithRotation('https://target-ecommerce-site.com');
}, 2000); // Adjust interval as needed
Bypassing Cloudflare’s security features requires a blend of technical expertise and strategic thinking. Utilizing dynamic proxies, emulating human behavior, managing browser fingerprints, handling CAPTCHAs, and rotating IPs are all critical tactics in this endeavor. Through Cloud API serves as a powerful tool in this toolkit, offering streamlined solutions for bypassing Cloudflare’s defenses.