Cloudflare is renowned for its advanced security features, including its anti-bot measures, WAF (Web Application Firewall), and CAPTCHAs. While these measures protect websites from malicious activities, they also pose challenges for legitimate automation tasks like web scraping and data collection. If you’re using browser fingerprint tools and seek a way to bypass Cloudflare’s defenses effectively, this tutorial is tailored for you. We will delve into using Node.js to integrate the Through Cloud API, enabling seamless bypass of Cloudflare’s 5-second shield, Turnstile CAPTCHA, and WAF protection.
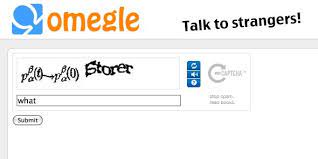
Introduction to Cloudflare’s Anti-Bot Measures
Cloudflare’s suite of security tools includes:
- 5-Second Shield: A delay mechanism to verify the legitimacy of traffic.
- Turnstile CAPTCHA: A challenge-response test to distinguish human users from bots.
- WAF: A firewall that protects against common threats by filtering HTTP traffic.
These features safeguard websites but can obstruct automation processes for data collectors. By integrating the Through Cloud API with Node.js, you can bypass these hurdles, enabling uninterrupted access to target sites.
Through Cloud API Overview
The Through Cloud API offers:
- Bypassing Cloudflare’s 5-Second Shield: Quickly skip the initial delay.
- Circumventing Turnstile CAPTCHA: Handle human verification checks effortlessly.
- Cloudflare WAF Bypass: Navigate through WAF protection without detection.
- HTTP API and Proxy Service: Access a global dynamic IP network and customize requests with specific parameters like Referer, User-Agent, and headless mode.
Why Use Node.js with Through Cloud API?
Node.js, with its asynchronous nature and robust ecosystem, is ideal for automation and web scraping tasks. Combining Node.js with the Through Cloud API allows you to build flexible and efficient solutions to bypass Cloudflare protections.
Prerequisites
Before we start, ensure you have the following:
- Node.js installed on your machine.
- npm (Node Package Manager) for managing dependencies.
- A Through Cloud API account.
Step-by-Step Integration
Let’s walk through setting up Node.js to work with the Through Cloud API for bypassing Cloudflare protections.
Step 1: Set Up Your Node.js Environment
- Install Node.js and npm: Download and install from nodejs.org.
- Initialize Your Project: Create a new project directory and initialize npm.bash复制代码
mkdir cloudflare-bypass cd cloudflare-bypass npm init -y
- Install Required Packages:bash复制代码
npm install axios npm install express npm install dotenv
- axios: For making HTTP requests.
- express: For setting up a local server if needed.
- dotenv: For managing environment variables.
Step 2: Configure Through Cloud API
- Register for Through Cloud API: Visit the Through Cloud API registration page and create an account.
- Obtain API Key: After registering, obtain your API key and necessary endpoint details.
- Create a
.env
File: Store your API key securely.bash复制代码touch .env
Add your API key:bash复制代码THROUGH_CLOUD_API_KEY=your_api_key_here
Step 3: Develop the Node.js Script
Create a script, cloudflare-bypass.js
, in your project directory.
3.1 Import Dependencies
require('dotenv').config();
const axios = require('axios');
3.2 Define Helper Functions
Create functions to make requests via the Through Cloud API.
const throughCloudApiUrl = 'https://api.throughcloud.com/bypass';
async function fetchPage(url) {
try {
const response = await axios.get(url, {
headers: {
'Authorization': `Bearer ${process.env.THROUGH_CLOUD_API_KEY}`,
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://example.com'
}
});
return response.data;
} catch (error) {
console.error('Error fetching page:', error);
}
}
3.3 Implement Cloudflare Bypass Functionality
Utilize Through Cloud API to bypass Cloudflare’s protections.
async function bypassCloudflare(targetUrl) {
try {
const response = await axios.post(throughCloudApiUrl, {
url: targetUrl
}, {
headers: {
'Authorization': `Bearer ${process.env.THROUGH_CLOUD_API_KEY}`
}
});
if (response.data.success) {
console.log('Bypassed Cloudflare:', response.data);
return response.data.content;
} else {
console.log('Failed to bypass Cloudflare:', response.data);
}
} catch (error) {
console.error('Error bypassing Cloudflare:', error);
}
}
Step 4: Fetch and Display Content
Create a function to fetch and log content from a target URL.
javascript复制代码async function main() {
const targetUrl = 'https://targetwebsite.com';
const content = await bypassCloudflare(targetUrl);
console.log('Fetched Content:', content);
}
main();
Step 5: Testing and Debugging
- Run the Script: Execute your script to test the integration.bash复制代码
node cloudflare-bypass.js
- Verify Results: Check the console for output and ensure that Cloudflare’s protections are bypassed successfully.
Advanced Configuration
Handling CAPTCHA Challenges
If you encounter CAPTCHAs, the Through Cloud API can handle these by processing the challenge-response.
async function handleCaptcha(targetUrl) {
try {
const response = await axios.post(`${throughCloudApiUrl}/captcha`, {
url: targetUrl
}, {
headers: {
'Authorization': `Bearer ${process.env.THROUGH_CLOUD_API_KEY}`
}
});
if (response.data.success) {
console.log('CAPTCHA solved:', response.data);
return response.data.content;
} else {
console.log('Failed to solve CAPTCHA:', response.data);
}
} catch (error) {
console.error('Error solving CAPTCHA:', error);
}
}
Dynamic IP Proxy Configuration
For large-scale scraping, configure dynamic IP proxies provided by Through Cloud API.
async function fetchWithProxy(targetUrl) {
try {
const response = await axios.get(targetUrl, {
proxy: {
host: 'dynamic_proxy_host',
port: 8080
},
headers: {
'Authorization': `Bearer ${process.env.THROUGH_CLOUD_API_KEY}`,
'User-Agent': 'Mozilla/5.0',
'Referer': 'https://example.com'
}
});
return response.data;
} catch (error) {
console.error('Error fetching with proxy:', error);
}
}
Conclusion
By integrating the Through Cloud API with Node.js, you can effectively bypass Cloudflare’s sophisticated defenses, including the 5-second shield, Turnstile CAPTCHA, and WAF. This approach leverages HTTP API and dynamic proxy services to provide seamless access to web content, facilitating tasks like web scraping, data collection, and automation.
Key Takeaways
- Through Cloud API enables bypassing Cloudflare’s anti-bot measures effectively.
- Node.js provides a flexible and efficient platform for integrating these bypass techniques.
- Dynamic IP proxies and customizable request parameters enhance the ability to mimic genuine user interactions, reducing detection risks.
Whether you’re a developer, data collector, or researcher, understanding how to bypass Cloudflare protections can significantly streamline your workflow. Using Through Cloud API with Node.js ensures you stay ahead in the realm of data access and automation, bypassing Cloudflare’s formidable barriers with ease.
For more details and to start using the Through Cloud API, visit Through Cloud API.