Navigating the intricate labyrinth of modern web security, especially with the formidable gatekeeper that is Cloudflare, is a challenge that often feels akin to unraveling a mystery. For data collection enthusiasts and Python developers, the quest to find the best Python Cloudflare scraper is not merely a technical task but a journey of discovery, innovation, and sometimes, frustration. The ultimate goal? To bypass Cloudflare and access the protected content without stumbling upon endless roadblocks.
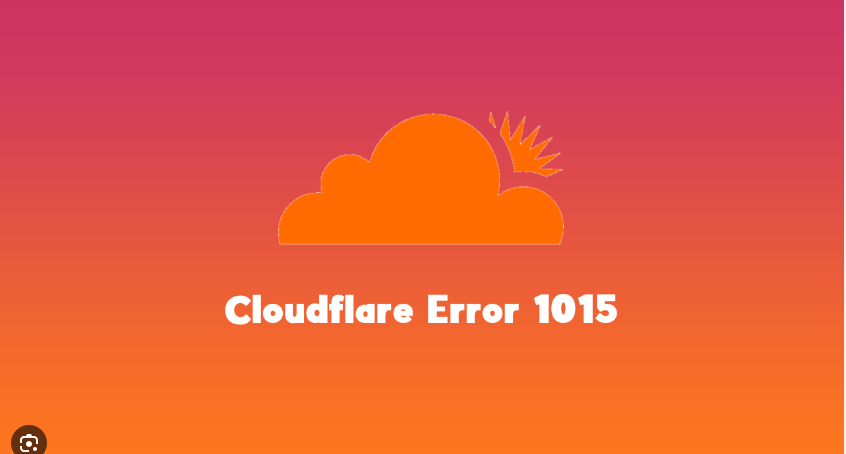
The Intrigue of Cloudflare’s Defenses
Imagine standing before a fortress. Cloudflare, the guardian of countless websites, is that fortress. It employs a range of sophisticated defenses designed to thwart bots and malicious actors, including:
- The 5-Second Shield (JavaScript Challenge): A temporary barricade requiring visitors to execute JavaScript to verify their legitimacy.
- Turnstile CAPTCHA: A vigilant sentry that blocks entry until a human proves they’re not a bot.
- Web Application Firewall (WAF): A protective barrier filtering out harmful traffic, making unauthorized data scraping nearly impossible.
These defenses make scraping data from Cloudflare-protected sites a Herculean task. Yet, the thrill of overcoming these barriers and reaching the data drives the relentless pursuit of the best Python scraper.
What Makes a Great Python Cloudflare Scraper?
To unearth the best Python Cloudflare scraper, consider the essential qualities:
- Capability to Bypass Cloudflare’s Shields: The scraper should gracefully handle Cloudflare’s JS challenges, CAPTCHA, and WAF.
- Support for Dynamic IP Rotation: To evade IP bans, dynamic IP proxies such as those provided by the Through Cloud API become invaluable.
- Headless Browser Support: Tools like Puppeteer and Selenium should be part of the arsenal to handle complex web pages and dynamic content.
- Customization Options: The ability to set custom User-Agent strings, HTTP headers, and other browser fingerprinting features is critical to mimic human behavior.
The Contenders: Python Tools to Tame Cloudflare
1. CloudScraper
CloudScraper is a trusted ally in the fight against Cloudflare’s initial defenses. It tackles the JavaScript challenge head-on, providing an uncomplicated way to scrape websites.
Installation:
pip install cloudscraper
Usage:
import cloudscraper
scraper = cloudscraper.create_scraper()
response = scraper.get('https://example.com')
print(response.text)
Why It’s Loved: CloudScraper is straightforward and effective for bypassing the initial JS challenge, making it a go-to tool for many developers.
Challenges: While adept at handling JavaScript challenges, CloudScraper might falter against CAPTCHAs and sophisticated WAF rules.
2. Selenium
Selenium provides a more holistic approach by automating browsers. It can execute JavaScript, handle complex page interactions, and mimic user behavior.
Installation:
bash复制代码pip install selenium
Usage:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
options = Options()
options.headless = True
service = Service('/path/to/chromedriver')
driver = webdriver.Chrome(service=service, options=options)
driver.get('https://example.com')
print(driver.page_source)
driver.quit()
Why It’s Beloved: Selenium’s ability to interact with dynamic content and render JavaScript makes it an invaluable tool for scraping.
Challenges: Its dependency on browser drivers and higher resource consumption can be limiting for some applications.
3. Pyppeteer
Pyppeteer, the Python port of Puppeteer, offers control over a headless Chrome browser, blending the power of a browser with the simplicity of Python.
Installation:
pip install pyppeteer
Usage:
import asyncio
from pyppeteer import launch
async def main():
browser = await launch()
page = await browser.newPage()
await page.goto('https://example.com')
content = await page.content()
print(content)
await browser.close()
asyncio.get_event_loop().run_until_complete(main())
Why It’s Adored: Pyppeteer excels at handling complex web interactions and rendering, making it perfect for dynamic and protected content.
Challenges: It can be resource-intensive and may require additional configuration on certain systems.
4. Through Cloud API
For those seeking a sophisticated solution, Through Cloud API stands out. It’s not just a scraper but a comprehensive toolset offering HTTP API access, dynamic IP proxies, and the ability to bypass multiple layers of Cloudflare’s defenses.
How to Use:
- Register and Obtain API Key: Sign up and get your API key.
- Configure API Requests: Use
requests
or any HTTP client to send requests via the API.
Installation:
pip install requests
Usage:
import requests
def bypass_cloudflare(url, api_key):
headers = {'Authorization': f'Bearer {api_key}'}
payload = {'url': url, 'method': 'GET'}
response = requests.post('https://throughcloudapi.com/bypass', headers=headers, json=payload)
return response.json()
api_key = 'YOUR_API_KEY'
url = 'https://example.com'
result = bypass_cloudflare(url, api_key)
print(result)
Why It’s Exceptional: Through Cloud API provides advanced capabilities for bypassing not just Cloudflare’s initial challenges but also CAPTCHAs and WAF, along with dynamic IP rotation.
Challenges: Reliance on an external service and potential costs associated with API usage.
Personal Insights and Best Practices
1. Merging Multiple Tools
In the pursuit of the best results, combining tools can be highly effective. For instance, using CloudScraper for initial requests and switching to Selenium or Pyppeteer for handling dynamic content ensures robust scraping.
Example Combination:
import cloudscraper
from selenium import webdriver
scraper = cloudscraper.create_scraper()
response = scraper.get('https://example.com')
if 'JS challenge' in response.text:
options = webdriver.ChromeOptions()
options.headless = True
driver = webdriver.Chrome(options=options)
driver.get('https://example.com')
content = driver.page_source
driver.quit()
else:
content = response.text
print(content)
2. Emulating Human Behavior
Mimicking real user behavior by customizing HTTP headers, User-Agent strings, and handling browser fingerprinting can significantly reduce detection risk.
Example Customization:
import requests
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://example.com'
}
response = requests.get('https://example.com', headers=headers)
print(response.text)
3. Utilizing Dynamic IP Proxies
Dynamic IP proxies prevent IP bans by rotating IP addresses, crucial for large-scale scraping operations. Through Cloud API offers a pool of dynamic IPs, ensuring continued access.
Example Proxy Integration:
import requests
def get_proxy(api_key):
headers = {'Authorization': f'Bearer {api_key}'}
response = requests.get('https://throughcloudapi.com/proxy', headers=headers)
return response.json()['proxy']
api_key = 'YOUR_API_KEY'
proxy = get_proxy(api_key)
session = requests.Session()
session.proxies = {'http': proxy, 'https': proxy}
response = session.get('https://example.com')
print(response.text)
The Journey Continues
Choosing the best Python Cloudflare scraper is a dynamic process involving experimentation and adaptation. Each tool, from CloudScraper to Through Cloud API, offers unique strengths and faces particular challenges. By understanding these tools and employing a combination of techniques, you can effectively bypass Cloudflare’s formidable defenses, turning what seems like a daunting task into a gratifying achievement.
As a data collection technician, this journey is not just about technology; it’s about the satisfaction of overcoming obstacles, the joy of unraveling complexities, and the relentless pursuit of knowledge and efficiency in the world of web scraping. So, equip yourself with these tools, refine your strategies, and embrace the thrill of the chase as you navigate the guarded gates of Cloudflare.