In the realm of web scraping, Cloudflare stands as a formidable guardian, employing various sophisticated mechanisms to prevent automated scripts from accessing data. For a web scraping developer, bypassing Cloudflare’s defenses can feel like a journey through a maze—one filled with dead ends, challenges, and occasional breakthroughs. This article will delve into how to bypass Cloudflare using Python, integrating Through Cloud API to simplify the process.
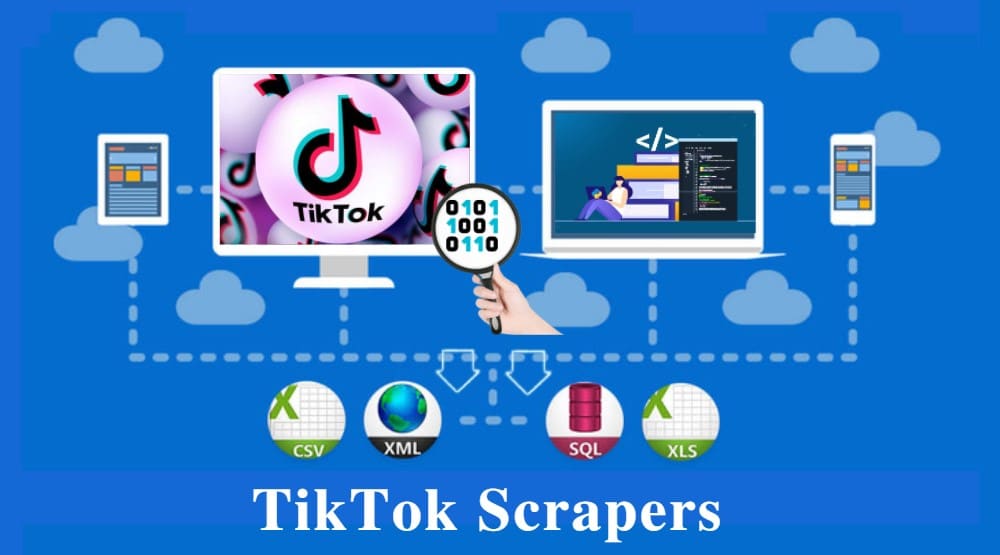
The Challenge of Cloudflare
Cloudflare’s protection mechanisms, such as the 5-second shield, Turnstile CAPTCHA, and Web Application Firewall (WAF), serve as significant hurdles for web scrapers:
- 5-Second Shield: This delays the request while Cloudflare assesses the visitor’s legitimacy through browser-based challenges.
- Turnstile CAPTCHA: Requires interaction to prove the user isn’t a bot, effectively blocking automated scripts.
- WAF: Inspects traffic for malicious activities, blocking requests that match known patterns of malicious behavior.
These layers of protection are designed to safeguard web servers from an onslaught of automated scraping, which often leads to high resource consumption or potential data breaches. For developers, bypassing these defenses is not only a technical challenge but also a game of cat and mouse.
Introducing Through Cloud API
Enter Through Cloud API, a powerful tool designed to navigate and bypass Cloudflare’s defenses seamlessly. Through Cloud API offers an HTTP API and a one-stop global dynamic S5 proxy service that simplifies bypassing Cloudflare’s 5-second shield, CAPTCHA challenges, and WAF protection.
Through Cloud API Overview
- Global Dynamic IP Pool: Over 350 million city-level dynamic IPs from more than 200 countries.
- Protocol Support: Supports HTTP and Socks5, ensuring flexibility in different scenarios.
- Browser Fingerprinting: Customize headers, User-Agent, Referer, and handle headless browsing features.
Bypassing Cloudflare with Python: A Step-by-Step Guide
Step 1: Setting Up Through Cloud API
- Register for Through Cloud API: Create an account and obtain your API key. This key will be used to authenticate your requests.
- Integrate API into Your Script: Through Cloud API offers an HTTP interface that you can incorporate into your Python scripts.
Step 2: Preparing Your Python Environment
To start, ensure you have the necessary libraries installed. You can use requests
for making HTTP requests and BeautifulSoup
for parsing HTML content:
bash复制代码pip install requests beautifulsoup4
Step 3: Configuring Your Script
Below is a sample Python script that integrates Through Cloud API to bypass Cloudflare:
import requests
# Through Cloud API configuration
proxy = {
"http": "http://username:[email protected]:1080",
"https://username:[email protected]:1080"
}
# Target URL
url = "https://target-website.com"
# Custom headers to simulate a real browser
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.82 Safari/537.36",
"Referer": "https://example.com"
}
# Sending the request through Through Cloud API
response = requests.get(url, headers=headers, proxies=proxy)
# Handling the response
if response.status_code == 200:
print("Successfully bypassed Cloudflare!")
print(response.text)
else:
print(f"Failed to bypass Cloudflare. Status code: {response.status_code}")
Explanation
- Proxy Configuration: Configure the proxy to route requests through Through Cloud API, which handles bypassing Cloudflare.
- Custom Headers: Adding headers like User-Agent and Referer helps mimic a real browser, reducing the chances of detection.
- Request Handling: Sends the request and checks the response status to determine if the bypass was successful.
Step 4: Handling CAPTCHA Challenges
To handle Turnstile CAPTCHA, Through Cloud API simplifies the process by managing CAPTCHA challenges in the background:
# Target URL with CAPTCHA
captcha_url = "https://target-website.com/captcha-protected"
# Sending the request through Through Cloud API
response = requests.get(captcha_url, proxies=proxy)
# Handling the response
if response.status_code == 200:
print("Successfully bypassed CAPTCHA!")
print(response.text)
else:
print(f"Failed to bypass CAPTCHA. Status code: {response.status_code}")
Step 5: Navigating WAF Protection
Cloudflare’s WAF scrutinizes request patterns to block suspicious activities. Through Cloud API dynamically adjusts IP and request patterns to avoid detection:
# Target URL with WAF protection
waf_url = "https://target-website.com/waf-protected"
# Sending the request through Through Cloud API
response = requests.get(waf_url, proxies=proxy)
# Handling the response
if response.status_code == 200:
print("Successfully bypassed WAF!")
print(response.text)
else:
print(f"Failed to bypass WAF. Status code: {response.status_code}")
Diving Deeper: Advanced Techniques
Using Browser Fingerprinting
To enhance the realism of your requests, you can use browser fingerprinting techniques. This involves setting up various headers and request parameters that resemble genuine browser traffic:
import requests
# Through Cloud API configuration
proxy = {
"http": "http://username:[email protected]:1080",
"https://username:[email protected]:1080"
}
# Target URL
url = "https://target-website.com"
# Advanced headers to mimic browser behavior
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.82 Safari/537.36",
"Referer": "https://example.com",
"Accept": "text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8",
"Accept-Encoding": "gzip, deflate, br",
"Accept-Language": "en-US,en;q=0.5",
"Connection": "keep-alive",
"Upgrade-Insecure-Requests": "1"
}
# Sending the request
response = requests.get(url, headers=headers, proxies=proxy)
# Handling the response
if response.status_code == 200:
print("Successfully bypassed Cloudflare with advanced headers!")
print(response.text)
else:
print(f"Failed to bypass Cloudflare. Status code: {response.status_code}")
Utilizing Headless Browsers
For more complex tasks, consider using headless browsers that simulate real user interactions. Tools like Selenium
can be combined with Through Cloud API to bypass Cloudflare while interacting with dynamic content:
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
# Through Cloud API configuration
proxy = "http://username:[email protected]:1080"
# Configure Selenium to use Through Cloud proxy
chrome_options = Options()
chrome_options.add_argument(f'--proxy-server={proxy}')
chrome_options.add_argument('--headless')
chrome_options.add_argument('--disable-gpu')
chrome_options.add_argument('--window-size=1920,1080')
# Path to your ChromeDriver
chrome_driver_path = '/path/to/chromedriver'
# Initialize the browser
browser = webdriver.Chrome(executable_path=chrome_driver_path, options=chrome_options)
# Target URL
url = "https://target-website.com"
# Visit the page
browser.get(url)
# Output page source
print(browser.page_source)
# Close the browser
browser.quit()
Explanation
- Headless Mode: Runs Chrome in headless mode to avoid opening a visible browser window, suitable for automated scripts.
- Proxy Integration: Routes traffic through Through Cloud API to handle Cloudflare’s defenses.
- WebDriver Configuration: Sets up ChromeDriver to interact with web pages like a real user.
Ethical Considerations
While bypassing Cloudflare’s security measures might be technically feasible, it’s imperative to adhere to ethical guidelines:
- Respect Websites: Avoid overloading servers with excessive requests.
- Follow Legal Protocols: Ensure your activities comply with legal standards and the terms of service of the websites you interact with.
- Use Responsibly: Bypass techniques should be used for legitimate purposes, such as research or testing.
Practical Applications
Bypassing Cloudflare can be beneficial in several scenarios:
- Data Aggregation: Collect data from multiple sources for analysis or research.
- Market Intelligence: Monitor competitors’ pricing and product availability in real-time.
- SEO Analysis: Gather SEO metrics from various websites to improve search engine performance.
Conclusion
Bypassing Cloudflare using Python and Through Cloud API transforms the challenge of web scraping into a manageable task. By leveraging dynamic IPs, customizable request parameters, and advanced browser fingerprinting techniques, you can navigate through Cloudflare’s 5-second shield, CAPTCHA, and WAF with ease. Remember, while it’s crucial to overcome technical barriers, ethical considerations must guide your actions. Through Cloud API empowers you to approach your scraping goals with both technical efficiency and ethical integrity.
In your journey as a web scraper, each successful request through Cloudflare feels like a small triumph. With tools like Through Cloud API, you’re not just scraping data—you’re overcoming obstacles and advancing your capabilities in the intricate dance between scrapers and defenses.