In the vast, interconnected digital world, access to information is a key asset. For data collection technicians, this access often means traversing the virtual barriers erected by security systems like Cloudflare. While these barriers are designed to safeguard websites, they also pose significant challenges to those who need legitimate, automated access. In this tutorial, we’ll delve into effective strategies for bypassing Cloudflare’s CAPTCHA challenges and WAF protections using proxy power and advanced API solutions.
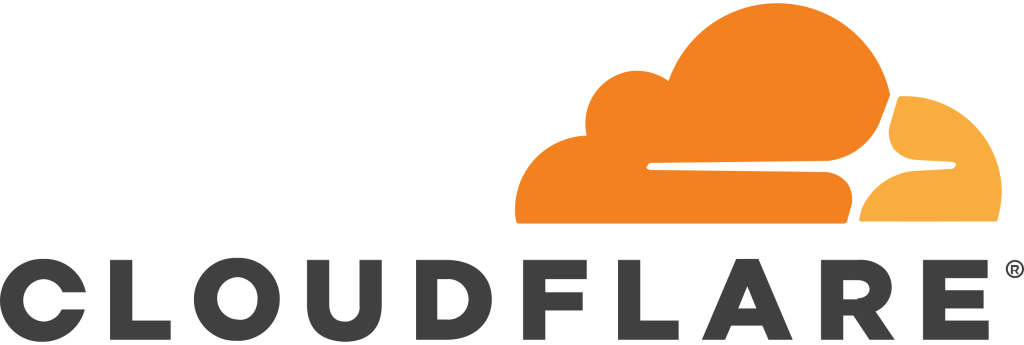
Understanding Cloudflare’s Defense Mechanisms
Before diving into bypassing techniques, it’s essential to understand the mechanisms you’re up against. Cloudflare deploys multiple layers of security to protect websites from automated threats:
- 5-Second Shield: A delay mechanism that challenges automated systems by imposing a waiting period.
- WAF (Web Application Firewall): A defense system that inspects incoming requests for suspicious patterns.
- Turnstile CAPTCHA: An advanced CAPTCHA system that verifies human presence through complex challenges.
These systems are effective in thwarting unauthorized access, but for legitimate data collection, they can be a formidable obstacle.
The Role of Proxies in Bypassing Cloudflare
Proxies act as intermediaries between your data collection tools and the target website. They mask your actual IP address, allowing you to avoid detection and access data more freely. Here’s how proxies can be leveraged to bypass Cloudflare’s defenses:
1. Dynamic IP Rotation
By using a dynamic IP proxy, you can continually change your IP address. This is akin to switching disguises while navigating through a guarded area, making it difficult for Cloudflare to track and block your requests.
Implementation Using Through Cloud API:
Through Cloud API provides access to a global dynamic IP proxy network with over 350 million city-level IPs across more than 200 countries. This vast pool allows for effective IP rotation, helping you evade detection.
Steps to Implement:
- Register and Set Up: Sign up for a Through Cloud API account and set up your access.
- API Integration: Integrate the API into your data collection scripts.
- IP Rotation Configuration: Use the provided interface addresses and request parameters to configure dynamic IP rotation.
Example Code Snippet:
import requests
def fetch_with_proxy(url):
# Replace with your API endpoint and access token
api_endpoint = "https://api.throughcloud.com/proxy"
headers = {
"Authorization": "Bearer your_access_token",
"Referer": "your_referer_url",
"User-Agent": "your_user_agent"
}
response = requests.get(api_endpoint, headers=headers, params={"target": url})
return response.content
url = "https://targetwebsite.com"
data = fetch_with_proxy(url)
print(data)
This snippet demonstrates a basic setup for fetching data through a dynamic proxy, with headers and request parameters tailored to evade Cloudflare’s tracking.
2. Geolocation Matching
Cloudflare uses geolocation data to detect suspicious activity. If your requests originate from multiple, far-apart regions in a short period, it raises red flags. Using proxies that match the geographical location of legitimate users can help you avoid this.
Implementation Using Through Cloud API:
Through Cloud API allows you to select proxies based on geographic locations, ensuring your requests appear to originate from expected regions.
Steps to Implement:
- Select Location: Choose the geographical region for your proxy IPs through the API settings.
- Integrate and Configure: Use the API to fetch proxies from the selected region and integrate this into your request handling.
Example Code Snippet:
def fetch_with_geo_proxy(url, location):
api_endpoint = "https://api.throughcloud.com/proxy"
headers = {
"Authorization": "Bearer your_access_token",
"Referer": "your_referer_url",
"User-Agent": "your_user_agent"
}
response = requests.get(api_endpoint, headers=headers, params={"target": url, "location": location})
return response.content
url = "https://targetwebsite.com"
data = fetch_with_geo_proxy(url, "New York")
print(data)
This code fetches data through a proxy from a specified location, aligning your request’s apparent origin with the target website’s expected traffic patterns.
3. Custom Headers and User-Agent Strings
Cloudflare analyzes request headers and User-Agent strings to detect automation. Crafting these headers to mimic genuine browser requests can help you blend in with legitimate traffic.
Implementation Using Through Cloud API:
Through Cloud API allows you to set custom headers, including the User-Agent string, Referer, and other fingerprinting details, making your automated requests indistinguishable from those of real users.
Steps to Implement:
- Define Headers: Create a set of headers that closely match those of popular web browsers.
- Configure API Requests: Integrate these headers into your API requests.
Example Code Snippet:
def fetch_with_custom_headers(url):
api_endpoint = "https://api.throughcloud.com/proxy"
headers = {
"Authorization": "Bearer your_access_token",
"Referer": "https://legitimatewebsite.com",
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36"
}
response = requests.get(api_endpoint, headers=headers, params={"target": url})
return response.content
url = "https://targetwebsite.com"
data = fetch_with_custom_headers(url)
print(data)
This snippet demonstrates how to set custom headers in your proxy requests to avoid detection.
Advanced Techniques for Bypassing Cloudflare CAPTCHAs
While proxies can help mask your identity, bypassing CAPTCHAs requires more advanced techniques. Here’s how to approach this challenge:
1. Headless Browser Automation
A headless browser simulates a real user’s interaction with a website without a graphical interface. This allows you to render JavaScript, solve CAPTCHAs, and navigate complex pages like a real browser would.
Tools and Libraries:
- Puppeteer: A Node.js library for controlling headless Chrome.
- Selenium: A powerful tool for web automation, supporting multiple browsers.
- Playwright: A versatile browser automation library supporting multiple browser engines.
Steps to Implement:
- Set Up Headless Browser: Choose a headless browser tool (e.g., Puppeteer) and set it up in your environment.
- Navigate and Solve CAPTCHAs: Use the tool to navigate to the target website, wait for CAPTCHA challenges, and solve them programmatically.
Example Code Snippet (Puppeteer):
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
await page.setExtraHTTPHeaders({
'Referer': 'https://legitimatewebsite.com',
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
});
await page.goto('https://targetwebsite.com');
await page.waitForSelector('.captcha-selector'); // Adjust selector based on actual CAPTCHA element
// Code to solve CAPTCHA goes here
const content = await page.content();
console.log(content);
await browser.close();
})();
This code sets up Puppeteer to navigate a website, handle headers, and potentially solve CAPTCHA challenges.
2. CAPTCHA Solving Services
For more complex CAPTCHAs, consider using third-party solving services that employ human solvers or advanced AI to handle CAPTCHA challenges.
Popular CAPTCHA Solving Services:
- 2Captcha: A widely used service that solves CAPTCHAs using human solvers.
- Anti-Captcha: An automated service for solving CAPTCHAs, including image and text-based challenges.
Steps to Implement:
- Sign Up for a Service: Choose a CAPTCHA solving service and sign up for an account.
- Integrate API: Use the service’s API to submit CAPTCHA challenges and receive solutions.
Example Code Snippet (2Captcha):
import requests
def solve_captcha(captcha_image_url):
api_key = "your_2captcha_api_key"
response = requests.post(
"http://2captcha.com/in.php",
data={"key": api_key, "method": "base64", "body": captcha_image_url}
)
captcha_id = response.text.split('|')[1]
return captcha_id
def get_captcha_solution(captcha_id):
api_key = "your_2captcha_api_key"
response = requests.get(
f"http://2captcha.com/res.php?key={api_key}&action=get&id={captcha_id}"
)
return response.text
captcha_image_url = "data:image/jpeg;base64,..." # Base64-encoded image of CAPTCHA
captcha_id = solve_captcha(captcha_image_url)
solution = get_captcha_solution(captcha_id)
print(f"Captcha Solution: {solution}")
This Python snippet integrates 2Captcha to solve CAPTCHA challenges using a base64-encoded image of the CAPTCHA.