In the era of data-driven decision-making, accessing web data efficiently and ethically is paramount. However, Cloudflare’s comprehensive security measures, including the 5-second shield, Turnstile CAPTCHA, and WAF (Web Application Firewall), can pose significant challenges for data technicians. This guide explores various techniques to bypass Cloudflare and provides practical insights for leveraging these methods effectively.
Understanding Cloudflare’s Protections
Cloudflare’s robust security suite includes:
- 5-Second Shield: A brief delay that checks incoming traffic.
- Turnstile CAPTCHA: A challenge-response mechanism to differentiate bots from humans.
- WAF: Filters and blocks malicious HTTP traffic, providing an additional security layer.
These measures protect websites but can obstruct legitimate automation tasks such as data collection, web scraping, and bot management.
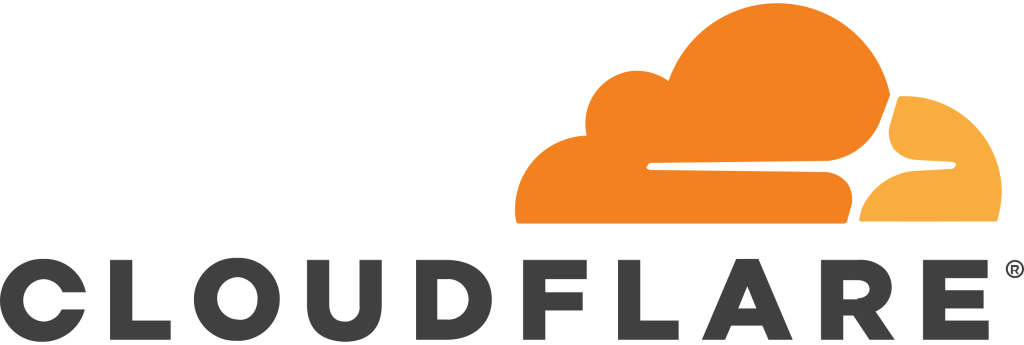
Why Bypass Cloudflare?
Data technicians need to bypass Cloudflare to:
- Access restricted web content for analysis or aggregation.
- Automate data collection without manual intervention.
- Ensure uninterrupted workflows for data-dependent applications.
Let’s delve into the methods to bypass Cloudflare’s protections, from basic approaches to advanced solutions like the Through Cloud API.
Basic Methods for Bypassing Cloudflare
1. Mimicking Legitimate Browsing
Browser fingerprinting is Cloudflare’s way of recognizing genuine user activity. To bypass Cloudflare, mimic typical browser behavior:
- User-Agent Spoofing: Use common browser User-Agents to disguise bot traffic as regular browsing.
- Referer Header: Set Referer headers to indicate traffic origins from expected sources.
Here’s a basic example using Node.js:
const axios = require('axios');
const fetchPage = async (url) => {
const response = await axios.get(url, {
headers: {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://example.com'
}
});
console.log(response.data);
};
fetchPage('https://targetwebsite.com');
Challenges
- Static Headers: Over-reliance on static headers can be detected.
- Behavioral Analysis: Cloudflare analyzes browsing patterns, making simple header spoofing less effective.
2. Rotating IP Addresses
Using a pool of IP addresses prevents Cloudflare from associating multiple requests with a single IP.
- Proxy Services: Employ residential or data center proxies to distribute requests across various IPs.
- Dynamic IP Rotation: Regularly change IP addresses to evade detection.
const axios = require('axios');
const proxyList = [
{ host: 'proxy1.example.com', port: 8080 },
{ host: 'proxy2.example.com', port: 8080 }
];
const fetchPageWithProxy = async (url) => {
const proxy = proxyList[Math.floor(Math.random() * proxyList.length)];
const response = await axios.get(url, {
proxy: {
host: proxy.host,
port: proxy.port
}
});
console.log(response.data);
};
fetchPageWithProxy('https://targetwebsite.com');
Challenges
- Proxy Quality: Low-quality proxies can be blacklisted.
- Cost: High-quality residential proxies can be expensive.
Advanced Methods for Bypassing Cloudflare
3. Browser Automation with Headless Browsers
Headless browsers simulate real browsing activity without a graphical interface, providing a more sophisticated way to bypass Cloudflare.
- Tools: Puppeteer and Playwright are popular headless browser frameworks.
- JavaScript Execution: These tools execute JavaScript, mimicking real user interactions.
const puppeteer = require('puppeteer');
const fetchPageWithHeadlessBrowser = async (url) => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(url);
const content = await page.content();
console.log(content);
await browser.close();
};
fetchPageWithHeadlessBrowser('https://targetwebsite.com');
Challenges
- Resource Intensive: Running headless browsers consumes more resources.
- Detection: Advanced bot detection systems may still recognize headless browsers.
4. Using the Through Cloud API
Through Cloud API is a specialized solution that integrates HTTP API and global dynamic IP proxy services to bypass Cloudflare’s security measures comprehensively.
Core Features
- Bypassing the 5-Second Shield: Skips the initial delay efficiently.
- Circumventing Turnstile CAPTCHA: Handles CAPTCHA challenges seamlessly.
- Cloudflare WAF Bypass: Navigates WAF protection using dynamic IPs and tailored request parameters.
Getting Started with Through Cloud API
- Register and Obtain API Key: Create an account on Through Cloud API and get your API key.
- Install Dependencies: Use axios for HTTP requests.bash复制代码
npm install axios dotenv
- Configure Environment Variables: Store your API key in a
.env
file.bash复制代码THROUGH_CLOUD_API_KEY=your_api_key_here
- Implement Through Cloud API in Node.js:
require('dotenv').config();
const axios = require('axios');
const throughCloudApiUrl = 'https://api.throughcloud.com/bypass';
const bypassCloudflare = async (url) => {
const response = await axios.post(throughCloudApiUrl, {
url: url
}, {
headers: {
'Authorization': `Bearer ${process.env.THROUGH_CLOUD_API_KEY}`
}
});
console.log(response.data);
};
bypassCloudflare('https://targetwebsite.com');
Advanced Configuration
Handling CAPTCHA Challenges: Use the CAPTCHA-solving capabilities of Through Cloud API.
const solveCaptcha = async (url) => {
const response = await axios.post(`${throughCloudApiUrl}/captcha`, {
url: url
}, {
headers: {
'Authorization': `Bearer ${process.env.THROUGH_CLOUD_API_KEY}`
}
});
console.log(response.data);
};
solveCaptcha('https://captcha-protected-site.com');
Dynamic IP Proxy Configuration: Utilize the dynamic IP proxy network provided by Through Cloud API for large-scale scraping.
const fetchWithDynamicProxy = async (url) => {
const response = await axios.get(url, {
proxy: {
host: 'dynamic_proxy_host',
port: 8080
},
headers: {
'Authorization': `Bearer ${process.env.THROUGH_CLOUD_API_KEY}`,
'User-Agent': 'Mozilla/5.0'
}
});
console.log(response.data);
};
fetchWithDynamicProxy('https://targetwebsite.com');
5. Handling JavaScript Rendering
Many modern websites rely heavily on JavaScript for content rendering. To effectively bypass Cloudflare and gather data from such sites:
- Use Headless Browsers: Execute JavaScript and collect dynamically loaded content.
- Through Cloud API: Utilize its built-in JavaScript rendering capabilities to simplify data collection.
const fetchDynamicContent = async (url) => {
const response = await axios.post(`${throughCloudApiUrl}/render`, {
url: url
}, {
headers: {
'Authorization': `Bearer ${process.env.THROUGH_CLOUD_API_KEY}`
}
});
console.log(response.data);
};
fetchDynamicContent('https://js-heavy-site.com');
Combining Methods for Robust Solutions
By integrating multiple methods, you can build a resilient system to bypass Cloudflare. For instance:
- Combine IP Rotation with Browser Automation: Use proxies with headless browsers to mask traffic patterns and mimic genuine user activity.
- Integrate Through Cloud API with Existing Workflows: Enhance existing data collection scripts by incorporating Through Cloud API’s advanced bypass techniques.
Best Practices and Ethical Considerations
While bypassing Cloudflare can facilitate data collection, adhere to ethical guidelines:
- Respect Terms of Service: Ensure your activities comply with the target site’s terms.
- Rate Limiting: Implement rate limits to avoid overwhelming servers.
- Data Privacy: Handle collected data responsibly, respecting user privacy and data protection laws.
Personal Insights
In my experience as a data technician, combining traditional methods with advanced tools like Through Cloud API offers the most effective approach to bypass Cloudflare protections. While basic techniques can address simple challenges, sophisticated measures are essential for handling modern security mechanisms effectively. The flexibility and robustness of the Through Cloud API make it an invaluable asset in my toolkit, especially for large-scale and complex data collection tasks.
Conclusion
Bypassing Cloudflare requires a blend of techniques tailored to the specific challenges posed by its protections. From mimicking legitimate browsing to leveraging advanced solutions like the Through Cloud API, data technicians can develop effective strategies to access web content seamlessly. By integrating these methods and adhering to ethical practices, you can enhance your data collection capabilities and ensure smooth, efficient workflows.